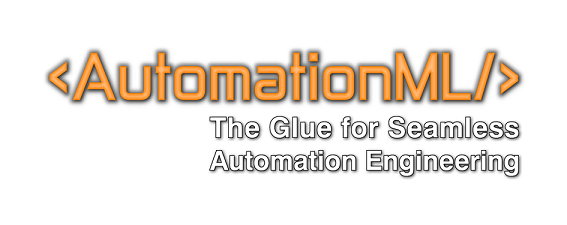 | ObjectWithAttributesSetAttributeValue(IObjectWithAttributes, String, Double) Method |
Modifies the attribute with the specified name from the attribute collection of the CAEX object, using
the provided double value. If no attribute with that name exists, it is created.
Sets the AttributeDataType to xs:double. To change existing attributes it is recommended to use
indexers (see examples below).
Namespace: Aml.Engine.CAEX.ExtensionsAssembly: Aml.Engine (in Aml.Engine.dll) Version: 3.2
Syntaxpublic static AttributeType SetAttributeValue(
this IObjectWithAttributes objWithAttr,
string attName,
double attValue
)
<ExtensionAttribute>
Public Shared Function SetAttributeValue (
objWithAttr As IObjectWithAttributes,
attName As String,
attValue As Double
) As AttributeType
public:
[ExtensionAttribute]
static AttributeType^ SetAttributeValue(
IObjectWithAttributes^ objWithAttr,
String^ attName,
double attValue
)
Parameters
- objWithAttr IObjectWithAttributes
- parent object
- attName String
- attribute name. This can be a path expression, if the attributes already exist
- attValue Double
- attribute value of type double
Return Value
AttributeTypeThe modified attribute
Usage Note
In Visual Basic and C#, you can call this method as an instance method on any object of type
IObjectWithAttributes. When you use instance method syntax to call this method, omit the first parameter. For more information, see
Extension Methods (Visual Basic) or
Extension Methods (C# Programming Guide).
Remarks
To modify an existing attribute value it is recommended to use an indexer.
Example
For example:
InternalElementType ie = GetInternalElementFromDocument();
ie.SetAttributeValue ("Length", 22.0);
results in a new added Attribute to the InternalElement with the name "Length" having the value 22.0 and the AttributeDataType xs:double.
InternalElementType ie = GetInternalElementFromDocument();
ie.SetAttributeValue ("Length", 24.0);
ie.Attribute["Length"].AttributeValue=24.0;
results in a changed attribute value of the InternalElements attribute "Length".
Example with nested attributes and path defined indexer:
InternalElementType ie = GetInternalElementFromDocument();
ie.SetAttributeValue ("Size/With", 10.0);
ie.SetAttributeValue ("Size/Hight", 20.0);
ie.Attribute["Size/With"].AttributeValue=10.0;
ie.Attribute["Size/Hight"].AttributeValue=20.0;
ie.Attribute["Size","With"].AttributeValue=10.0;
ie.Attribute["Size","Hight"].AttributeValue=20.0;
results in a changed attribute value of the InternalElements attribute "Size"
and its value parts.
See Also